ProxyJump를 사용하여 프록시 서버나 점프 호스트를 통해 서버에 접속해보자
환경
- Linux
- SSH
ProxyJump
목적
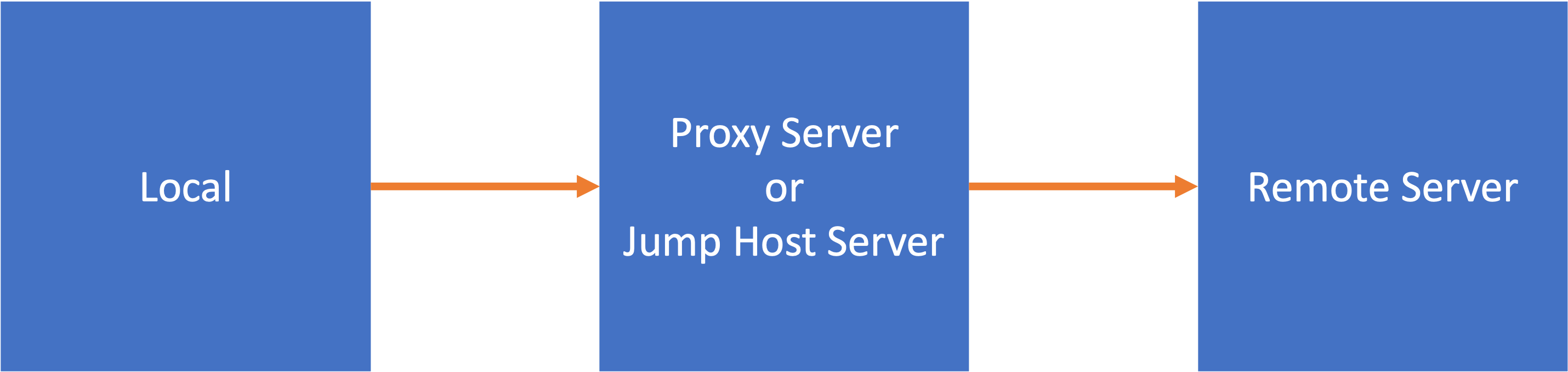
보안을 위해 실서버 접속시 프록시 혹은 점프 호스트를 통해서만 접속할 수 있는 구조가 많이 사용되는데 번거롭게 ssh 명령어를 각각 사용하는것보다 ProxyJump를 사용하면 한번의 설정으로 보다 쉽게 접속이 가능하다.
사용법
- 형태
Host [proxy server name]
HostName [proxy or jump host domain or ip address]
Port [port number]
User [username]
IdentityFile [proxy or jump host identity key file]
Host [remote server name]
HostName [server domain or ip address]
Port [port number]
User [username]
IdentityFile [server identity key file]
ProxyJump [proxy server name]
예제
Local
->proxy-server
->remote-server
- 접속시 사용하는
IdentityFile
은 둘 다Local
에 있어야 한다. - 아래는 예제 용도로 운영시에는 아래와 달리
Local
->proxy-server
는 외부망으로 연결되어 있고proxy-server
->remote-server
는 내부망으로만 연결되어있는 경우가 많다.
Host proxy-server
HostName 192.168.64.3
Port 22
User twpower
IdentityFile ~/.ssh/proxy-server
Host remote-server
HostName 192.168.64.4
Port 22
User twpower
IdentityFile ~/.ssh/remote-server
ProxyJump proxy-server
- 바로
remote-server
로 접속
ssh remote-server